(or, Column scope and binding order in subqueries)
I keep seeing this in all sorts of places. People getting an unexpected result when working with a subquery, typically an IN subquery, and assuming that they’ve found a bug in SQL Server.
It’s a bug alright, in that developer’s code though.
Let’s see if anyone can spot the mistake.
We’ll start with a table of orders.
CREATE TABLE Orders (
OrderID INT IDENTITY PRIMARY KEY,
ClientID INT,
OrderNumber VARCHAR(20)
)
There would be more to it in a real system, but this will do for a demo. We’re doing some archiving of old orders, of inactive clients. The IDs of those inactive clients have been put into a temp table
CREATE TABLE #TempClients (
ClientD INT
);
And, to check before running the actual delete, we run the following:
SELECT * FROM dbo.Orders
WHERE ClientID IN (SELECT ClientID FROM #TempClients)
And it returns the entire Orders table. The IN appears to have been completely ignored. At least the query was checked before doing the delete, that’s saved an unpleasant conversation with the DBA if nothing else.
Anyone spotted the mistake yet?
It’s a fairly simple one, not easy to see in passing, but if I test the subquery alone it should become obvious.
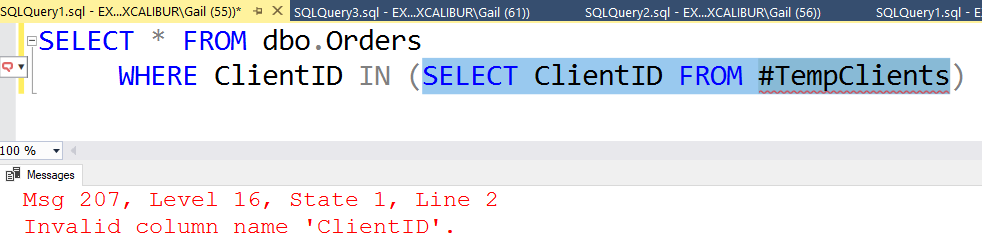
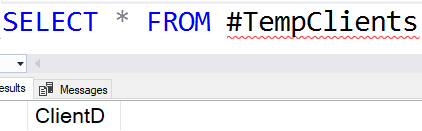
The column name in the temp table is missing an I, probably just a typo, but it has some rather pronounced effects.
The obvious next question is why the select with the subquery in it didn’t fail, after all, the query asks for ClientID from #TempClients, and there’s no such column. However there is a ClientID column available in that query, and it’s in the Orders table. And that’s a valid column for the subquery, because column binding order, when we have subqueries, is first to tables within the subquery, and then, if no match is found, to tables in the outer query.
It has to work this way, otherwise correlated subqueries would not be possible. For example:
SELECT c.LegalName,
c.HypernetAddress
FROM dbo.Clients AS c
WHERE EXISTS (SELECT 1 FROM dbo.Shipments s WHERE s.HasLivestock = 1 AND c.ClientID = s.ClientID)
In that example, c.ClientID explicitly references the Client table in the outer query. If I left off the c., the column would be bound to the ClientID column in the Shipments table.
Going back to our original example…
SELECT * FROM dbo.Orders
WHERE ClientID IN (SELECT ClientID FROM #TempClients)
When the query is parsed and bound, the ClientID column mentioned in the subquery does not match any column from any table within the subquery, and hence it’s checked against tables in the outer query, and it does match a column in the orders table. Hence the query essentially becomes
SELECT * FROM dbo.Orders
WHERE ClientID IN (SELECT dbo.Orders.ClientID FROM #TempClients)
Which is essentially equivalent to
SELECT * FROM dbo.Orders
WHERE 1=1
This is one reason why all columns should always, always, always, be qualified with their tables (or table aliases), especially when there are subqueries involved, as doing so would have completely prevented this problem.
SELECT * FROM dbo.Orders o
WHERE o.ClientID IN (SELECT tc.ClientID FROM #TempClients tc)
With the column in the subquery only allowed to be bound to columns within the #TempClients table, the query throws the expected column not found error.

And we’re no longer in danger of deleting everything from the orders table, as we would have if that subquery had been part of a delete and not a select.
Can’t agree more on always making sure that you qualify your column names with the table name/alias. The only time I honestly don’t bother if I’m only referencing a single table but as soon as two, or more, objects are being referenced it doesn’t make any sense to not add the qualifiers.
Plus it makes it far easier for someone who doesn’t know your schema to know where the data is coming from; which is always a great benefit when working in a team or hiring new people.
Pingback: The Importance of Aliasing in Subqueries – Curated SQL
Reminds me of OPTION EXPLICIT in VB. (So, why not have the same in SQL? 😉
What, forcing all columns to be qualified?